A short explanation for how to properly logout using PassportJs, Express-Session for single page web application. This short tutorial will share how to fully logout server side and clear the client side cookie as well.
The short answer:
/*** LOGOUT A USER ***/
app.post('/api/logout', (req, res, next) => {
res.clearCookie('connect.sid'); // clear the session cookie
req.logout(function(err) { // logout of passport
req.session.destroy(function (err) { // destroy the session
res.send(); // send to the client
});
});
});
A thorough explanation:
While the documentation for PassportJs and Express Session are relatively clear for login in a user, I could not find great documentation for how these all worked together to logout a user. Hence, I'm not sure if the above is the official and proper way to logout, and only arrived at this by much trial and error. My goal was to clear the session on both the server and client. So, I'll walk you through a short summary of my tests and findings! 🤓
Logging out with the PassportJs logout function
app.post('/logout', function(req, res, next){
req.logout(function(err) {
if (err) { return next(err); }
res.redirect('/');
});
});
PassportJs provides the example above, but this won't work for my single page web app, as I want to avoid using res.redirect('/')
. So below is my first re-write:
/*** LOGOUT A USER ***/
app.post('/api/logout', (req, res, next) => {
req.logout(function(err) {
console.log(err)
});
});
Using req.logout
will logout the user and clear the cookie on our database, but it does not clear that pesky cookie on the client. You can find this cookie by opening google developer console in your browser and clicking the applications
tab.
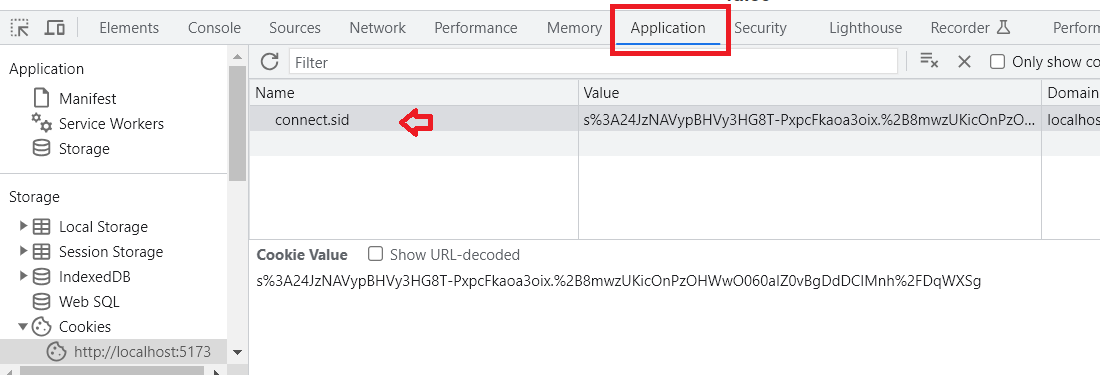
Adding ExpressJs Clear Cookie and sending
Next, I am adding res.clearCookie()
provided by ExpressJs. I am also creating an empty send response to update our client (see code comments below):
/*** LOGOUT A USER ***/
app.post('/api/logout', (req, res, next) => {
res.clearCookie('connect.sid'); // clear the cookie
req.logout(function(err) {
console.log(err)
res.send(); // send to the client
});
});
Interestingly, this also clears the cookie on the database, but just keeps updating our client side cookie to something new?
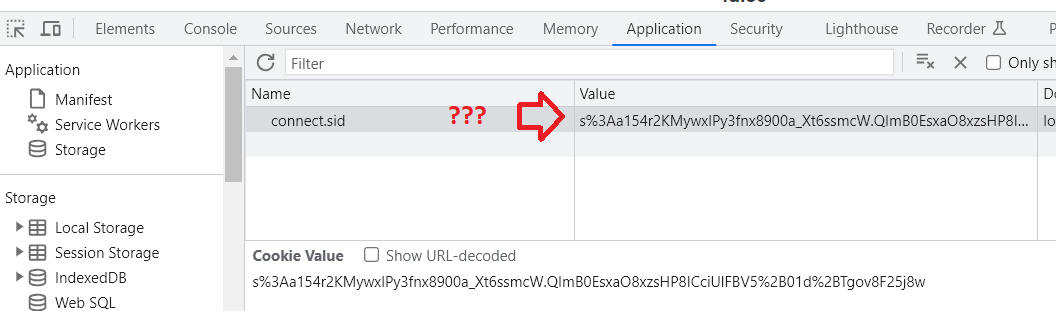
Finally, let's Destroy the Session in Express-Session
Express-session has a function to destroy our session. Let's then destroy our session prior to sending to the client, as shown in the commented code below.
/*** LOGOUT A USER ***/
app.post('/api/logout', (req, res, next) => {
res.clearCookie('connect.sid');
req.logout(function(err) {
console.log(err)
req.session.destroy(function (err) { // destroys the session
res.send();
});
});
});
After firing up our server again, we see that the above code clears the session in our database. It also clears our cookie client side (Hooray!).
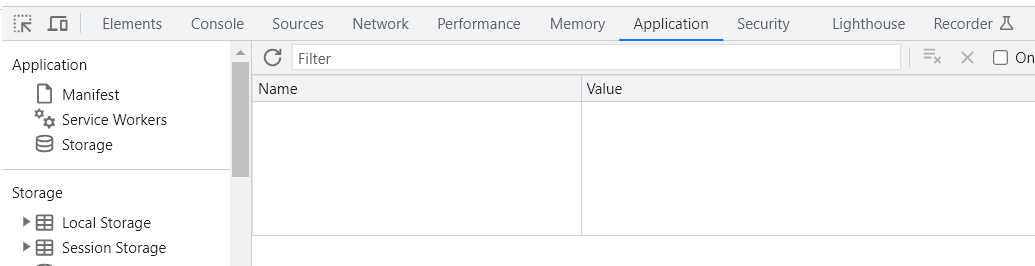